safeWallet
A wallet configurator for Safe Wallet which allows integrating the wallet with React
import { safeWallet } from "@thirdweb-dev/react";
const safeWalletConfig = safeWallet();
customize (optional)
safeWalletConfig
contains the default config for metadata and UI. you can optionally choose to override the defaults to customize the wallet. Learn more about these configs
const safeWalletConfig = safeWallet({ ... });
// override metadata
safeWalletConfig.meta.name = "..."; // change the name
safeWalletConfig.meta.iconURL = "..."; // change the icon
safeWalletConfig.meta.urls = {
// change urls to download the wallet on various platforms
android: "https://...",
ios: "https://...",
chrome: "https://...",
firefox: "https://...",
};
// override connection UI
safeWalletConfig.connectUI = SafeWalletConnectUI; // react component
// custom selection UI
safeWalletConfig.selectUI = SafeWalletSelectUI; // react component
Once the config is ready, you can use it with ConnectWallet
component or useConnect
hook as shown below
// add to ThirdwebProvider to add it in ConnectWallet's modal
<ThirdwebProvider supportedWallets={[safeWalletConfig]} clientId="your-client-id"/>;
// or use it with useConnect hook
const connect = useConnect();
connect(safeWalletConfig, { ... });
options
personalWallets (optional)
An array of personal wallets to show in ConnectWallet Modal for personal wallet selection
This is only relevant when connecting safeWallet with ConnectWallet component.
import {
safeWallet,
metamaskWallet,
coinbaseWallet,
walletConnect,
} from "@thirdweb-dev/react";
safeWallet({
// this is the default
personalWallets: [metamaskWallet(), coinbaseWallet(), walletConnect()],
});
recommended (optional)
Show this wallet as "recommended" in the ConnectWallet Modal.
paperWallet({
recommended: true,
});
Usage with ConnectWallet
To allow users to connect to this wallet using the ConnectWallet component, you can add it to ThirdwebProvider's supportedWallets prop.
import {
safeWallet,
metamaskWallet,
coinbaseWallet,
walletConnect,
} from "@thirdweb-dev/react";
<ThirdwebProvider
supportedWallets={[
safeWallet({
// this is the default
personalWallets: [metamaskWallet(), coinbaseWallet(), walletConnect()],
}),
]}
clientId="your-client-id"
>
<YourApp />
</ThirdwebProvider>;
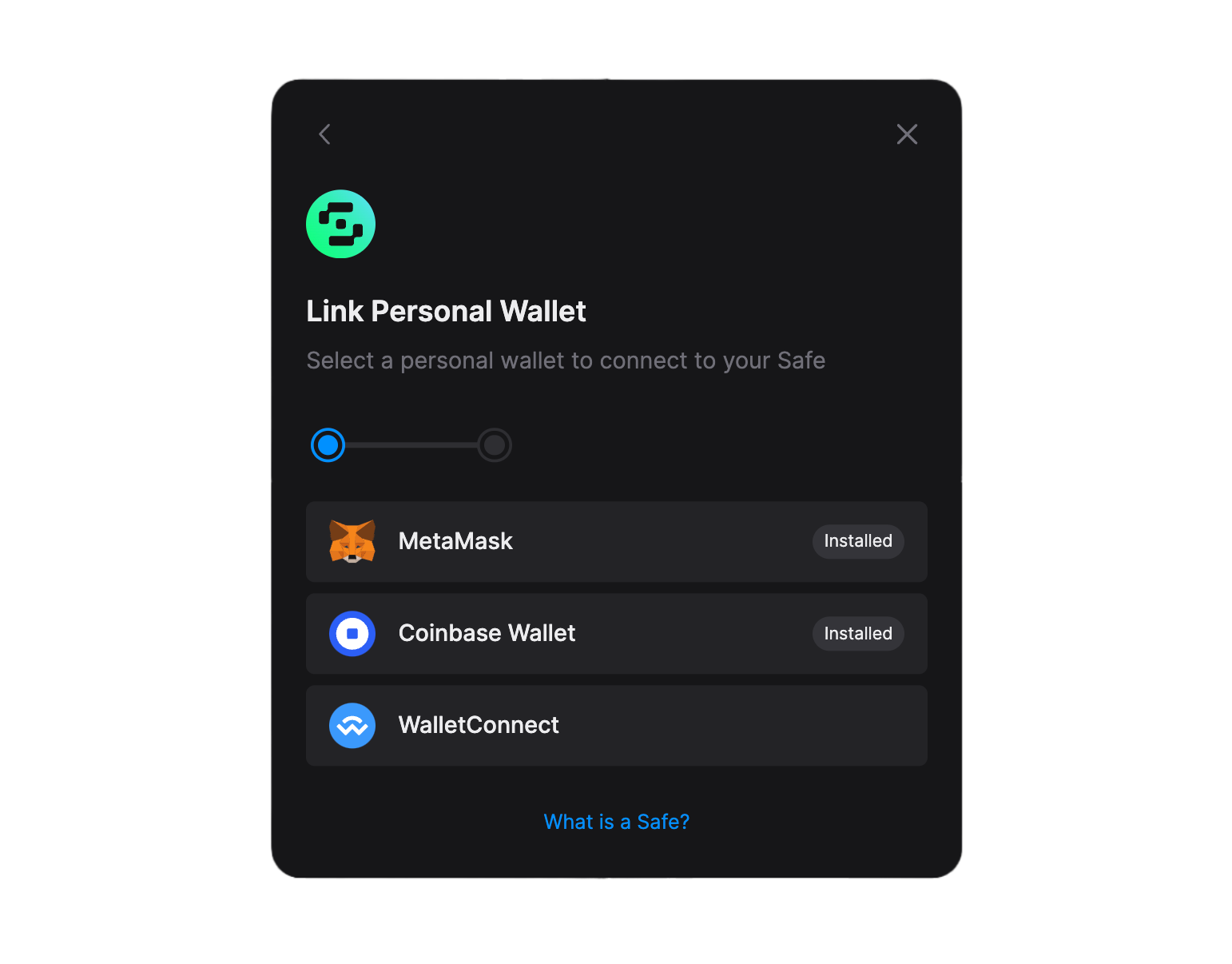
Usage with useConnect
you can use the useConnect
hook to programmatically connect to the wallet without using the ConnectWallet component.
The wallet also needs to be added in ThirdwebProvider's supportedWallets if you want the wallet to auto-connect on next page load.
You need to connect to a personal wallet first, You can use the useConnect hook to connect to a personal wallet first and then connect to the Safe Wallet. Make sure personal wallet is on the same network as the Safe Wallet.
const safeWalletConfig = safeWallet();
function App() {
const connect = useConnect();
const handleConnect = async () => {
await connect(safeWalletConfig, connectOptions);
};
return <div> ... </div>;
}
connectOptions
import type { Chain } from "@thirdweb-dev/chains";
import type { EVMWallet } from "@thirdweb-dev/wallets";
type ConnectOptions = {
chain: Chain;
personalWallet: EVMWallet;
safeAddress: string;
};
chain
Chain
object corresponding to the network that Safe Wallet is deployed to
personalWallet
The instance of a personal wallet that can sign transactions on the Safe.
safeAddress
Smart contract address of the Safe wallet.